Introduction :
In this blog, you will learn how to create a simple login using angular with bootstrap design.
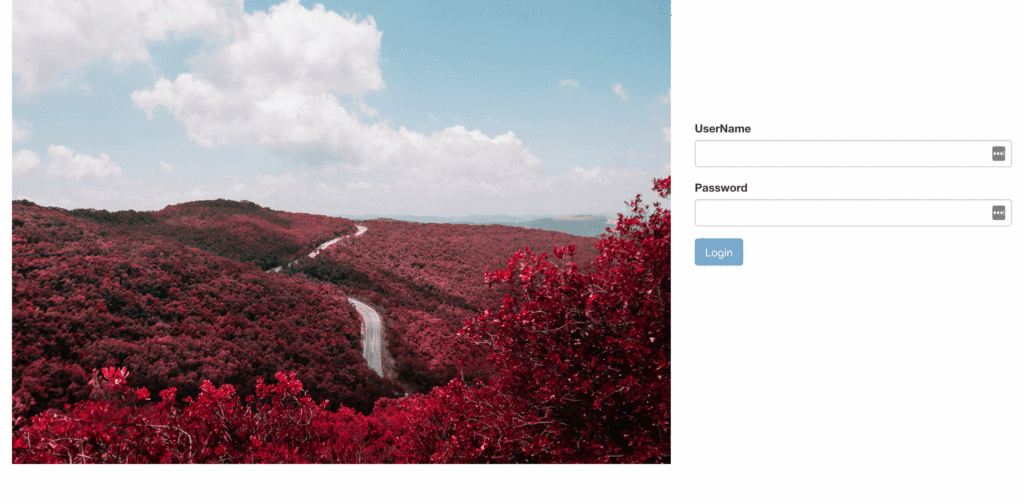
Explanation :
For creating a login, I use the angular forms module. For this example, I use template-driven forms. We will talk about the reactive form later. To use the form module, you have to include a form module in the app.module.ts file.
App.module.ts :
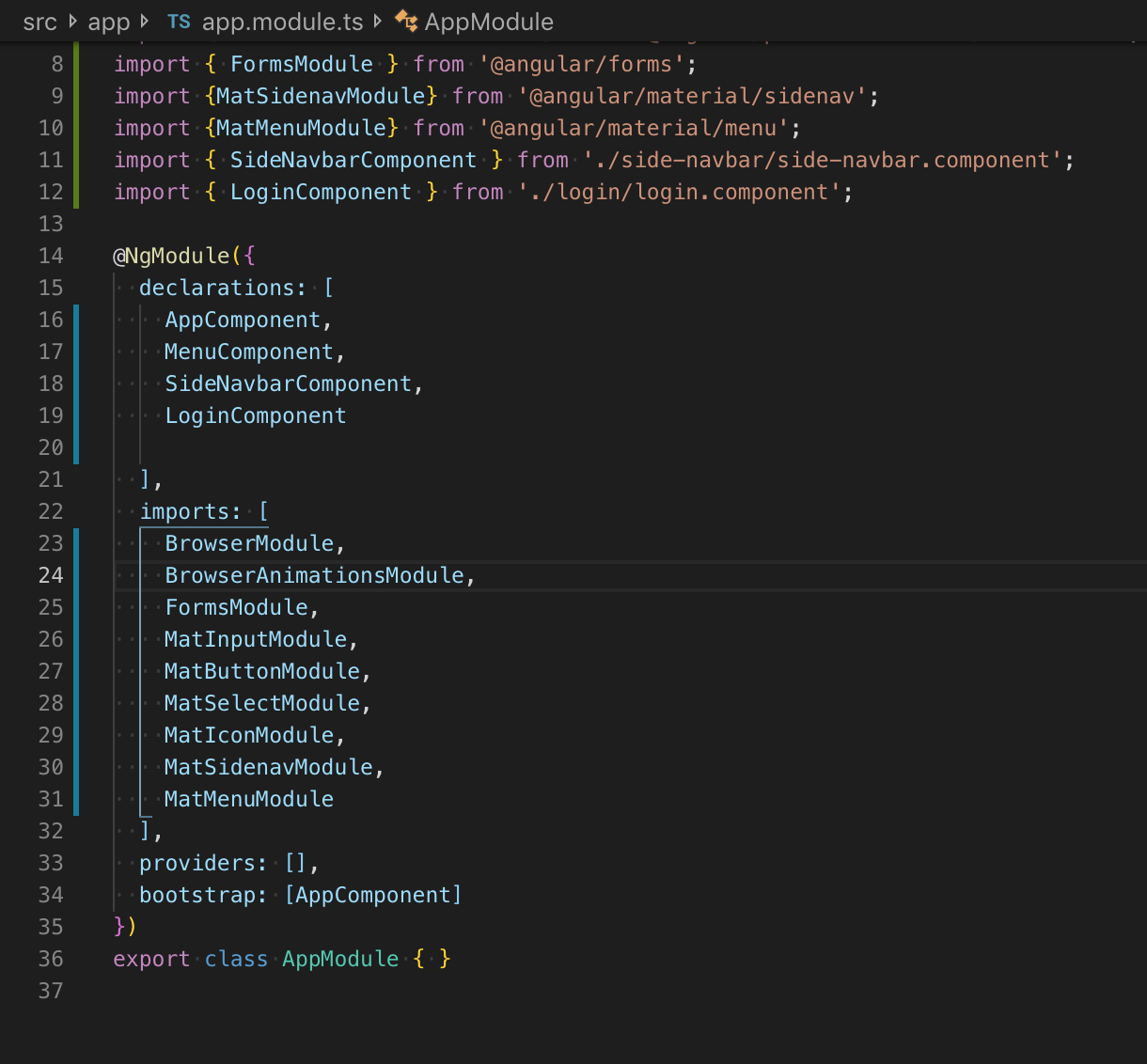
Create a login component by ng g c login.
This will create login.component.ts, login.component.ts and login.component.css.
First, we will see login.component.html.
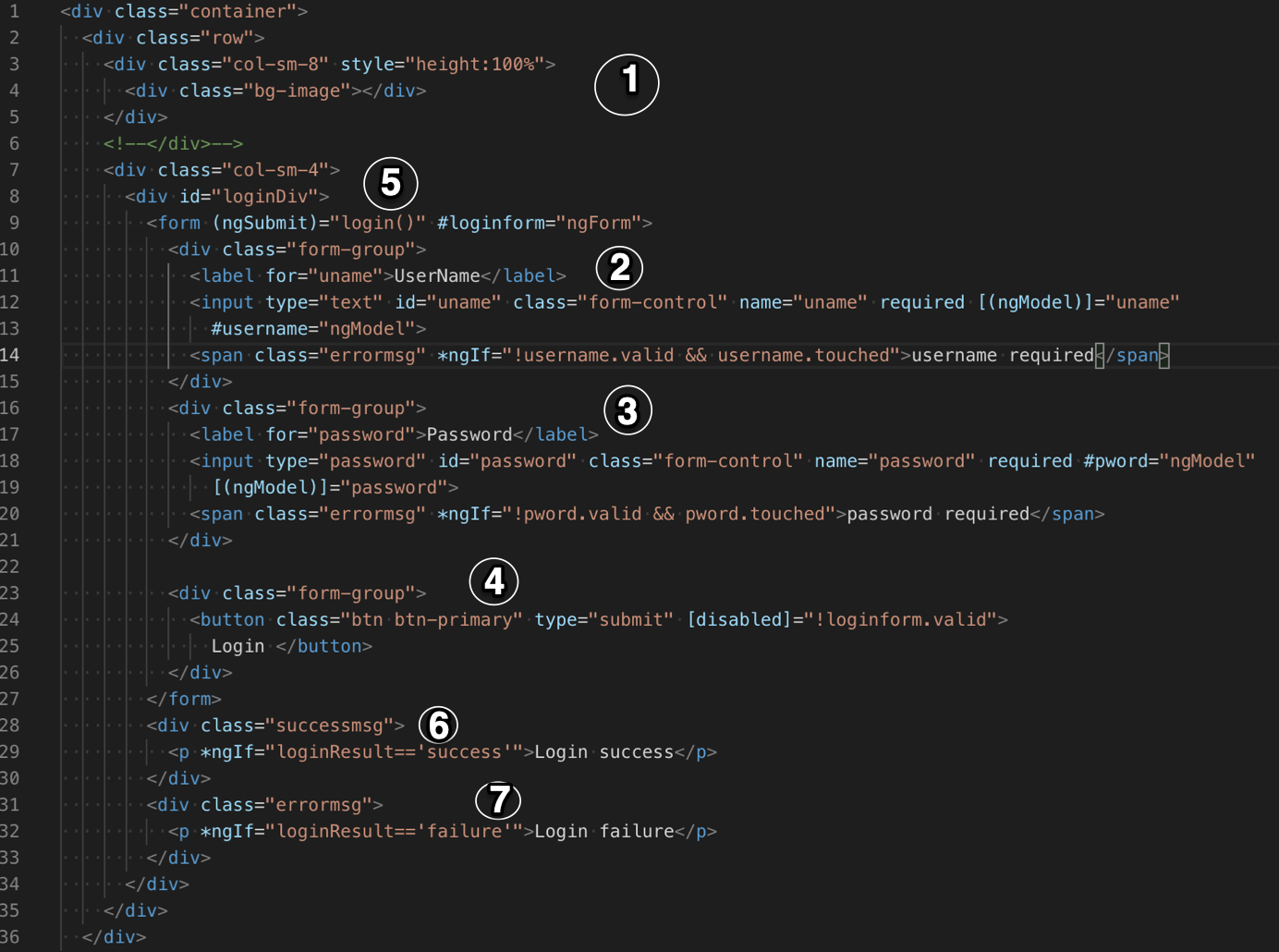
- In login.component .html add bootstrap container.Here we split column by col-sm-8 and col-sm-4. So with col-sm-8, we will have an image and in col-sm-4 we will have login div.
- Create loginDiv and inside that uname ,password input with button .form is HTML form element.”form-group ” is angular class .”form-control” used to declare the uname input element as form control . #username gives the reference of username. This is useful when we do the validation .
<span class="errormsg"
*ngIf="!username.valid && username.touched">
username required</span>
- And [(ngModel)]=”uname” is used for two day databinding .(two wany databinding means when you update the input text box value ,it will be automatically updated in the dom and typescript variable.
- Create a button with type submit and [disbaled] property is used to disable the button if the loginform is not valid. Loginform is the reference created for the form object ngFrom .
- Create a button with type submit and [disbaled] property is used to disable the button if the loginform is not valid. Loginform is the reference created for the form object ngFrom .
- ngSubmit=login() will submit the form control to typescript login() function and here #loginform is the reference for the from object ngForm.This ngForm is automatically created by angular and I have logged this form in typescript login.component.ts.
login.component.ts
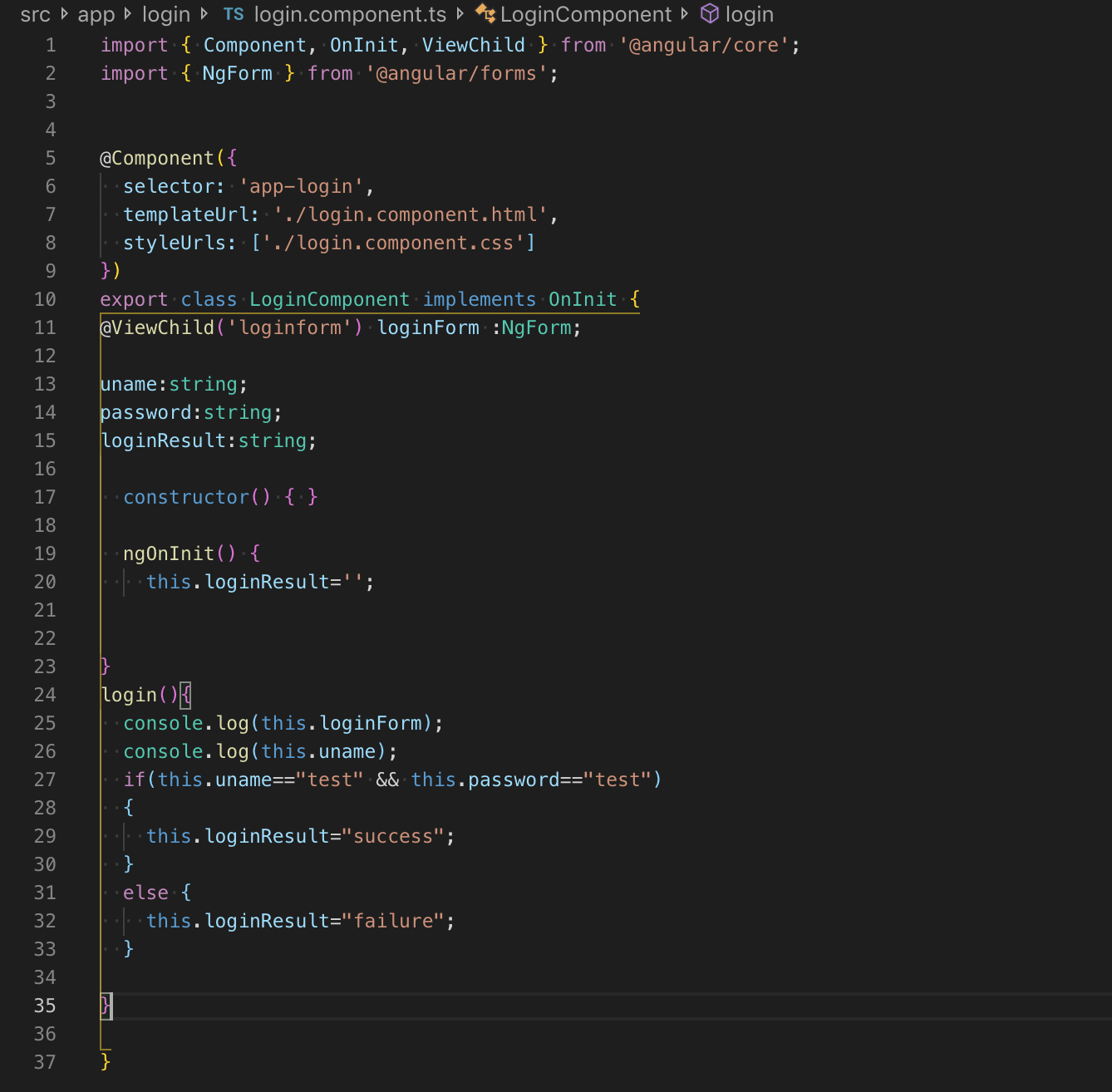
Here, this.loginFrom gives the below output.
NgForm printed in the console
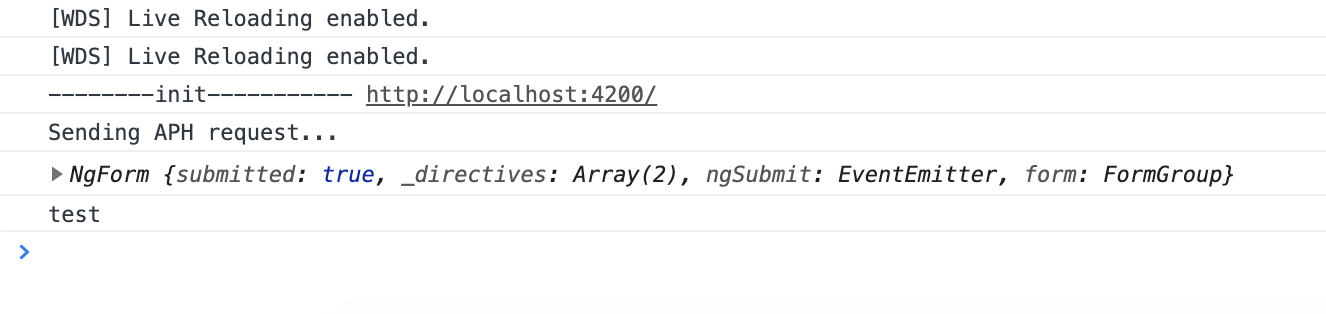
NgForm all properties
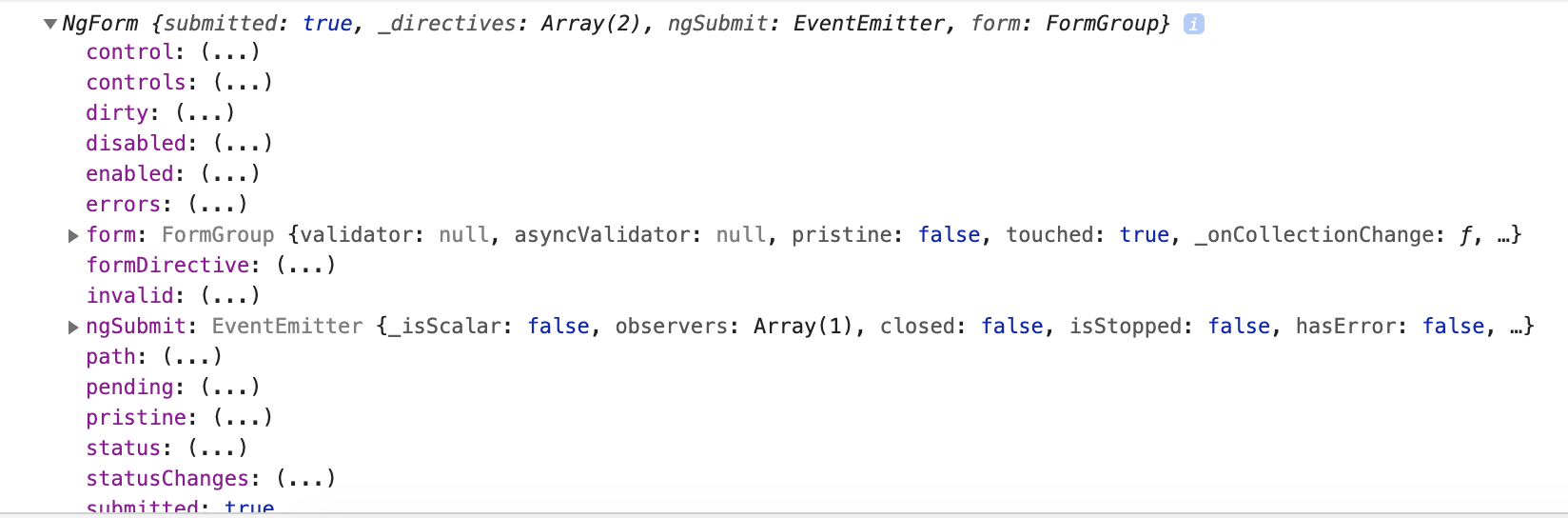
ng-form values uname and password
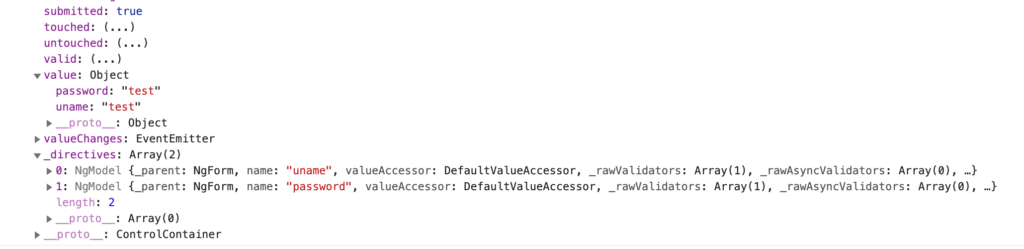
In login() function, we will check if the username and password are equal to test and if it is “test ” we will set a variable as success. This is just for this example. In real-time you will make a call to API and do this check.
Based on the “loginResult” variable we are displaying success message or error message.
login.component.css
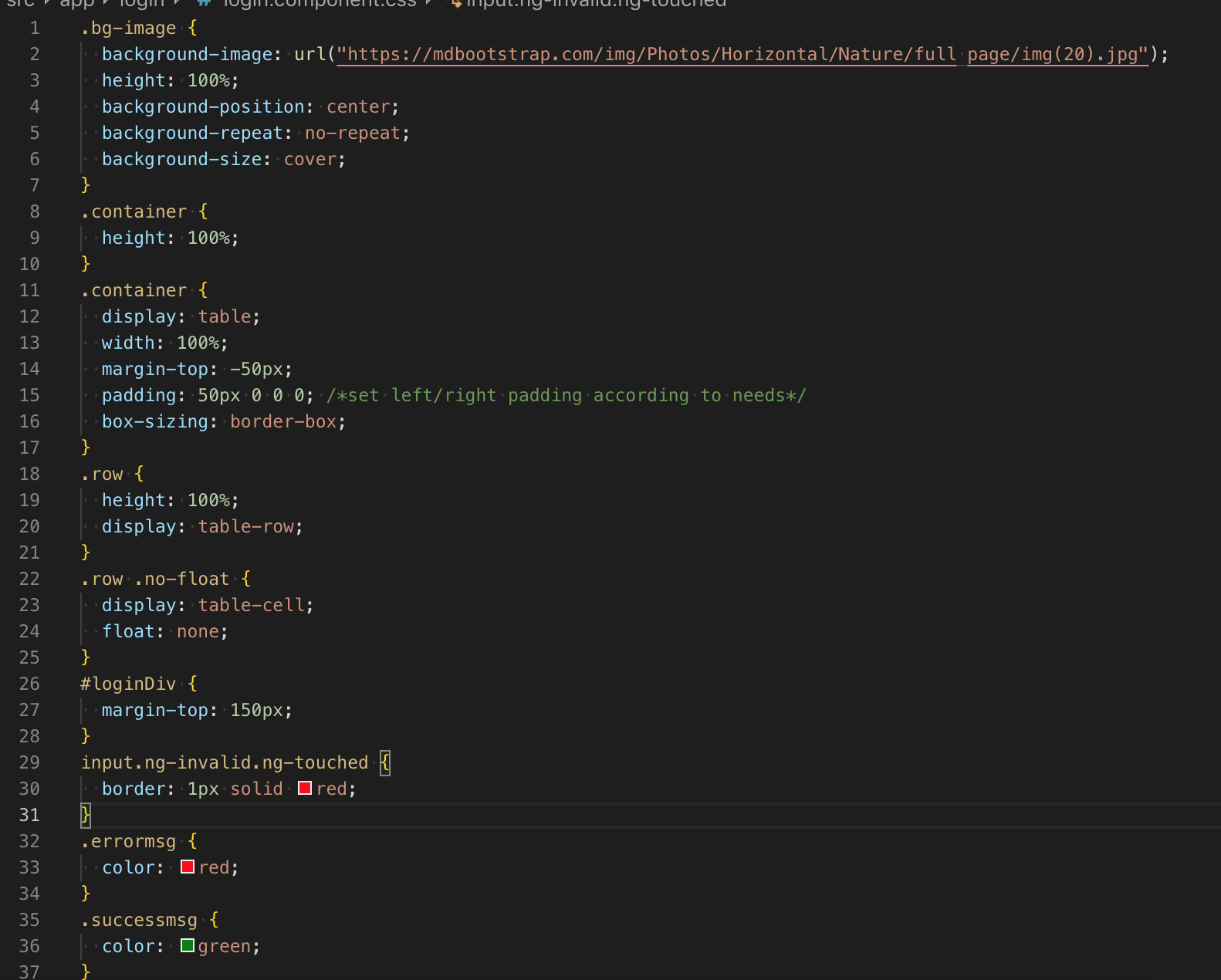